Lab 6: 15-Puzzle GUI
Due by the end of class
A 15-puzzle is a kind of puzzle made up of 15 tiles on a 4 × 4 grid. Thus, there are 15 tiles but 16 spaces, leaving one empty space. By moving one of the tiles surrounding the empty space to the empty space, the order of tiles can be changed. These tiles are typically numbered or have a picture printed on them such that one arrangement of the tiles puts all the numbers in ascending order or forms the picture. Thus, the goal of the puzzle is to move the tiles around until this ordering can be achieved.
A 15-puzzle is the name for this particular, common size of this puzzle, but an 8-puzzle is essentially the same, having 8 tiles on a 3 × 3 grid, and other sizes are possible as well.
The goal of this lab is to complete a playable GUI for a 15-puzzle using the Java Swing library. It should look something like the following, although the tiles might be randomized differently.
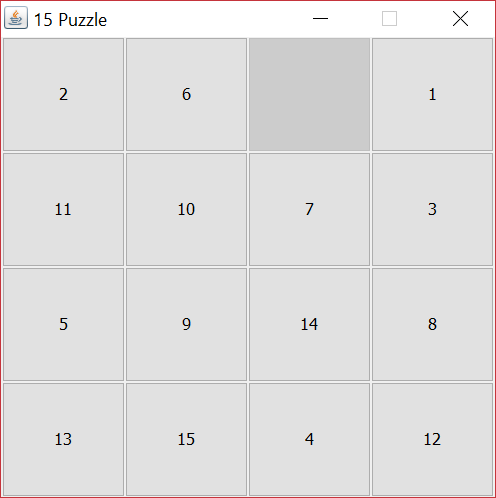
Specification
Create a project called Lab6
and create a package in your project called puzzle
. Download the following two files and add them to the puzzle
package.
Details
There are a whopping 20 TODO comments you must fill out inside the Puzzle
class, and it would be redundant to list them here. Try to understand everything you're doing.
The TODOs walk you through the process of representing the tiles in the GUI with 16 buttons (including an "empty" button with the number 0) and adding an ActionListener
to each one so that it moves correctly. The hard work of figuring out which neighboring button is empty is done for you by calling a method. Note that it's important to add the buttons in order from 1 up to SIZE*SIZE - 1
(15 for a 15-puzzle) and then add 0 as the last button. We take a solved puzzle and then scramble it rather than randomly placing tiles because it is possible to create unsolvable puzzles.
Successfully completing the Puzzle
class will result in a fully playable game that will announce when you have won.
By adjusting the size
variable in the main()
method, you can play a 3 × 3 puzzle known as an 8-puzzle. You might wish to do so for debugging purposes, since it's time-consuming to try to beat the full 15-puzzle.
Turn In
Turn in your code by uploading Puzzle.java
from the Lab6\src\puzzle
folder inside your workspace folder to Blackboard. Do not upload the entire project. I only want the Puzzle.java
file.
All work must be done individually. Never look at someone else's code. Please refer to the course policies if you have any questions about academic integrity. If you have trouble with the assignment, I am always available for assistance.