Lab 5: JOption Pain
Due by the end of class
The game of 20 Questions starts with the three categories of Animal, Vegetable, and Mineral and then allows a player to guess an object from a given category by asking a series of yes-or-no questions. When played competitively, the yes-or-no questions are limited to 20. Mathematicians will note that three categories and 20 questions, even when optimally chosen, will only allow 3,072 different items to be distinguished.
The program explored in this lab takes the role of the player, guessing the object that the user has in mind. It is pre-loaded with three objects, one for each category: a cardinal, a maple tree, and iron. However, if the user is thinking of something that is not contained in the tree of choices, the program will work through the available questions and eventually prompt the user to come up with a new question that distinguishes the new item from the previously guessed item. In this way, the game will learn new objects over time. Unfortunately, the objects learned will be lost every time the program is restarted. A more sophisticated program would store all of the objects in a file or a database that could persist between program runs.
Your goal, however, is only to add simple GUI elements that give information or ask questions and feed the responses back to the program. All of this GUI interaction will be done through the mechanism of JOptionPane
.
Specification
Create a project called Lab5
and create a package in your project called questions
. Download the following seven files and add them to the questions
package.
- Animal.java
- AnimalVegetableMineralQuestion.java
- Block.java
- Game.java
- Mineral.java
- Vegetable.java
- YesOrNoQuestion.java
Feel free to investigate the inheritance hierarchy used to build this game. However, the only file you need to edit is Game.java
. This file contains seven TODO comments. Each one of these comments must be replaced by a called to a static JOptionPane
method.
The following list explains what must be done for each of the TODO comments.
- Category question
- Yes-or-no question
- Is this what you are thinking of question
- Hooray message
- What's the name of your object question
- Enter a new question question
- Keep playing question
The first use of JOptionPane
should ask the user, "What are you thinking of?" It should allow the user to pick from the options given by the array categories
. It should look like the following.
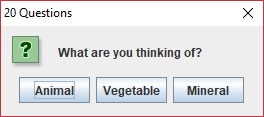
The second use of JOptionPane
should ask the user a yes-or-no question given by calling current.getText()
, which retrieves the text for the current Block
in the tree. It should look something like the following, although the question will change based on which Block
is currently selected.
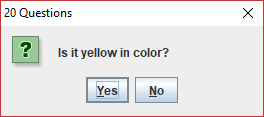
The third use of JOptionPane
should ask the user a yes-or-no question asking whether the object specified by current.getText()
is what you're thinking of. Note that the question should include the kind of object ("animal"
, "vegetable"
, or "mineral"
), which is stored in the kind
variable. It should look something like the following, although the question will change based on the guess being made.
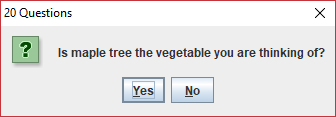
The fourth use of JOptionPane
should display a message saying something like, "Hooray! We guessed your animal." Whether the last word in the sentence is "animal," "vegetable," or "mineral" depends on the value in the kind
variable, like in the last section. It should look like the following, substituting the one of the three categories as appropriate.
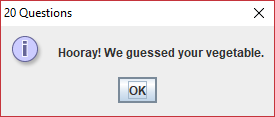
The fifth use of JOptionPane
is for when the game failed to guess the object. In that case, it's going to add a new object to the tree of objects. Thus, it must ask, "What's the name of your animal?" The last word in the sentence should again be "animal," "vegetable," or "mineral," depending on the value in the kind
variable. It should look like the following, substituting the one of the three categories as appropriate.
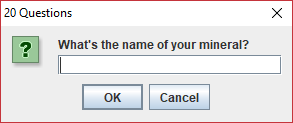
The sixth use of JOptionPane
is also for when the game failed to guess the object. After finding out the name of the new object, it must learn a question that can distinguish the new object from the last object reached. For example, the JOptionPane
method could request the user to, "Enter a question whose answer is 'yes' for gold but 'no' for iron." In general, the message will substitute the name of the new object for "gold" and the name of the old object for "iron." It should look like the following, with appropriate name substitutions.
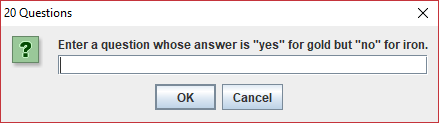
The seventh and final use of JOptionPane
simply asks, "Keep playing?" It expects a yes-or-no response and should look like the following.
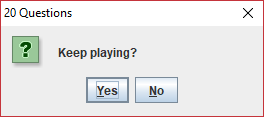
Hints
The only thing that you have to do for this lab is write seven different calls to JOptionPane
methods. However, there are a number of useful methods in this class, and many of them have several overloaded forms that take different numbers and kinds of arguments. Please consult the Java API page for JOptionPane
and Chapter 7 from the textbook.
To make the hunt for the right methods a little easier, I will confirm that you will need only the following methods. Most of these will be used more than once. Note: You will need to supply a title for every single method. Fortunately, a title is supplied for you in the TITLE
constant.
JOptionPane.showConfirmDialog()
JOptionPane.showInputDialog()
JOptionPane.showOptionDialog()
JOptionPane.showMessageDialog()
Turn In
Turn in your code by uploading Game.java
from the Lab5\src\questions
folder inside your workspace folder to Blackboard. Do not upload the entire project. I only want the Game.java
file.
All work must be done individually. Never look at someone else's code. Please refer to the course policies if you have any questions about academic integrity. If you have trouble with the assignment, I am always available for assistance.