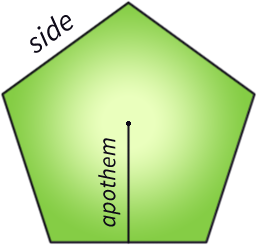
Project 1: Purchasing Polygonal Properties
Due by: Friday, September 8, 2023 at 11:59 p.m.
All of the properties on the Greek island of Polygos are shaped like regular polygons. A regular polygon is one whose sides are all the same length and whose angles are all the same as well, such as a square or an equilateral triangle.
Your program will help people calculate how much a given property would cost to buy. Cost is based on area because of the price of land as well as perimeter because of the cost of the fencing which must be put around all properties on Polygos.
Specification
Your program must first read in six pieces of data: two String
values, an int
value, and three double
values.
- First name
- Last name
- Sides the property has
- Side length in meters
- Property cost per square meter
- Fence cost per meter
From this data, you must compute the following seven values:
- Total area: the area covered by the polygon
- Total perimeter: the distance around the edge of the polygon in meters
- Length of apothem: the distance from the center of the polygon to the middle of one of its sides
- Interior angle: the interior angle at which sides of the polygon meet
- Cost of land: the property cost per square meter multiplied by the total area
- Cost of fencing: the fence cost per square meter multiplied by the total perimeter
- Total cost: the cost of land plus the cost of fencing
Then, your program must read in two additional pieces of data, a double
value and an int
value. These pieces of data assume that the user is taking out a loan for the total cost at a fixed interest rate over a set number of years.
- Annual interest rate
- Length of loan in years
Finally, your program must compute the following value:
- Total cost with interest: the total cost including loan interest
Once these values have been computed, they must be presented with formatting that exactly matches the sample output below.
Sample Execution
Below is sample output from the execution of the program. The user is prompted for his or her first name, last name, sides the property has, side length in meters, property cost per square meter, and fence cost per meter. User input is marked in green
, just as it is in IntelliJ. Note that this is only a sample. Users must be able to enter any name and any values for the property dimensions and costs.
After these values are read in, an appropriately named title for the table of property information, using the first initial and the last name of the user, is printed, followed by 64 asterisks on the next line. Then, the seven computed values of property information are printed on separate lines.
Then, the words Loan Information
are printed on a separate line, followed by 64 asterisks on the next line. The user is prompted for the annual interest rate and the length of the loan in years.
After these values are read in, the computed value of the total cost with interest is printed on a separate line.
Note: Your output must match exactly to the character to get full credit.
Welcome to the Polygonal Property Calculator! Enter your first name: Barry Enter your last name: Wittman Enter the sides the property has: 7 Enter side length in meters: 10.4 Enter property cost per square meter: $3.50 Enter fence cost per meter: $6.75 Property Information for B. Wittman **************************************************************** Total area: 393.044 square meters Total perimeter: 72.800 meters Length of apothem: 10.798 meters Interior angle: 128.571 degrees Cost of land: $1375.65 Cost of fencing: $491.40 Total cost: $1867.05 Loan Information **************************************************************** Enter annual interest rate: .05 Enter length of loan in years: 10 Total cost with interest: $3041.23
Equations
In order to compute the correct geometric values, there are several formulas you may find useful. In these equations the variable sides refers to the number of sides of the polygon, length refers to the length of a side, apothem is the length of the apothem, area is the total area of the polygon, perimeter is the perimeter of the polygon, and angle is the interior angle of the polygon.
- apothem = ½ length · cot(180° / sides)
- area = ½ sides · length · apothem
- perimeter = sides · length
- angle = 180° · (sides - 2)/sides
To compute the total cost with interest you will only need one equation. For this equation, cost is the total amount of money including interest, principal is the initial amount of money borrowed, rate is the annual interest rate, and years is the length of the loan in years.
- cost = principal · (1 + rate)years
These formulas use mathematical notation. Remember that steps must be taken to convert mathematical notation into equivalent Java syntax. In particular, dividing integers in mathematics can produce rational numbers. In Java, dividing two integers will always make another integer. Also, all trigonometric methods in the Java Math
class use radians as angle units, although the equations above all use degrees. You will need to convert the degrees to radians before using the Math.tan()
method.
Hints
Tabs
You might notice that the values all line up in a column. This occurs because tabs are being used for spacing. You can use the escape sequence \t
inside of a String
literal to print a tab. Most entries will require three tabs, but the two longest entries will only require one.
Decimals
All computed geometric values show exactly three places after the decimal point. All costs show exactly two places after the decimal point to represent cents. To get full points, your output must match. Use the System.out.format()
method to accomplish this formatting.
Turn In
Your IntelliJ project should be called Project1
, but the class you create inside should be called Polygon
. Upload the Polygon.java
file from the Project1\src
folder wherever you created your project to Blackboard.
All work must be submitted before Friday, September 8, 2023 at 11:59 p.m. unless you are going to use a grace day.
All work must be done individually. You may discuss general concepts with your classmates, but it is never acceptable for you to look at someone else's code. Please refer to the course policies if you have any questions about academic integrity. If you have trouble with the assignment, I am always available for assistance.
Grading
Your grade will be determined by the following categories, based largely on correctly computing four geometric values and four costs:
Category | Weight |
---|---|
Computing total area | 10% |
Computing total perimeter | 10% |
Computing length of apothem | 10% |
Computing interior angle | 10% |
Computing cost of land | 10% |
Computing cost of fencing | 10% |
Computing total cost | 10% |
Computing total costs with interest | 10% |
Matching output formatting exactly | 10% |
Coding style and comments | 10% |
Under no circumstances should any student look at the code written by another student. Tools will be used to detect code similarity automatically.
Code that does not compile will automatically score zero points.