Lab 13: Bright Ideas
Due by the end of class
The goal of this lab is to make a tool that can do a simple adjustment of the brightness of an image, making it either darker or lighter.
Specification
Create a project called Lab13
. Add a Brightness
class (with a main()
method) as well as a Picture
class. Delete everything inside the Picture
class and paste in the code from Picture.java.
Input
Inside of the main()
method in the Brightness
class, prompt the user to enter the name of the image file to work with. Read in this String
and create a new Picture
object using the file name in the constructor.
Then, prompt the user for a brightness level between 0 and 5 and read in this value as a double
.
Brightness
Create two nested for-loops that iterate through every pixel in the image (just like a 2D array, except that you have to use the get()
method with an x
and a y
parameter to get a pixel at a particular location).
Get the Color
value for each pixel. Then, extract the red, green, and blue values from the Color
object using the getRed()
, getGreen()
, and getBlue()
methods.
Multiply the red, green, and blue values by the brightness value entered by the user. If any of them are larger than 255, set them to 255. For example, if the user entered a brightness value of 1.6, the image will get brighter (values less than 1 will make it darker). So, a pixel with R, G, and B values of (35, 200, 18) would become (56, 255, 28).
Finally, once the new red, green, and blue values have been computed, set the current pixel to a new Color
made from the updated red, green, and blue values, rounded to the nearest integer using Math.round()
. You can create the Color
using a constructor. Then, you can set the pixel value to that Color
using the set()
method.
Output
After updating all the pixels in the for loops, call the show()
method once to show the image with adjusted brightness.
Here is an example of the user editing the image workstation.jpg
and changing it to a brightness of 2.6. Please try to match the output formatting exactly.
What picture would you like to edit? workstation.jpg How bright do you want your image? (0-5) 2.6
Below is the image before editing:
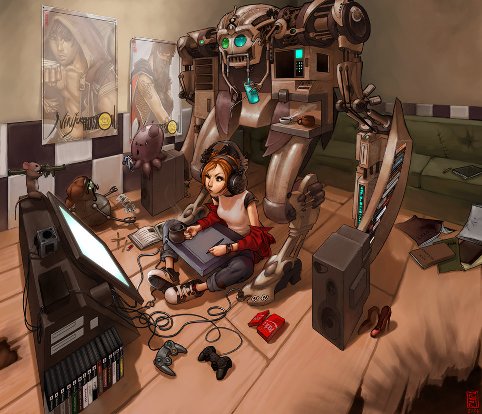
Below is the image after editing:
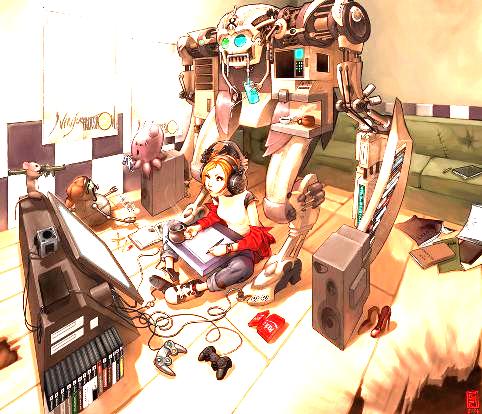
You can download the original image by right-clicking here and saving the target or the link. Alternatively, you should be able to download any image ending in .jpg or .png from the Internet and use that for testing.
Turn In
Turn in your code by uploading Brightness.java
from the Lab13\src
folder wherever you created your project to Blackboard. Do not upload the entire project. The Picture.java
file is unnecessary. I only want the Brightness.java
file.
All work must be done individually. Never look at someone else's code. Please refer to the course policies if you have any questions about academic integrity. If you have trouble with the assignment, I am always available for assistance.