Lab 12: Asteroids
Due by the end of class
The goal of this lab is to complete a couple of Java classes to finish the implementation for a simplified game of Asteroids. In the original game of Asteroids, the goal was to destroy asteroids, breaking them into smaller pieces whild avoiding the debris and surviving as long as possible. Unfortunately, the game for this lab only includes dodging the asteroids, not destroying them.
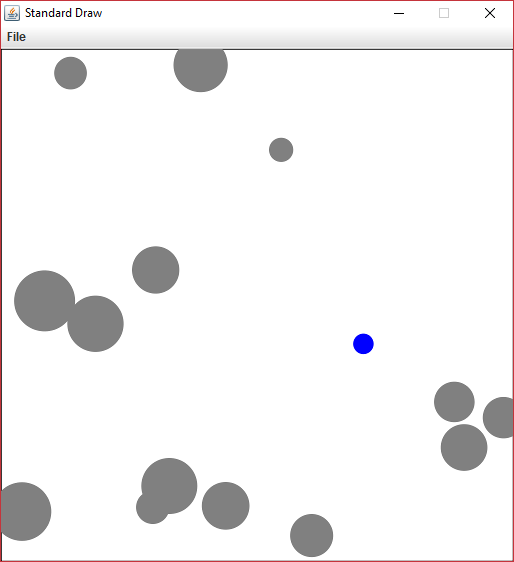
Specification
Create a project called Lab12
. Add the following classes: Asteroid
, Ship
, Simulator
, and StdDraw
. Delete everything inside of all the Java files you have just added.
Below are the instructions for each class file.
Asteroid
Paste the code from Asteroid.java
into your Asteroid
class file.
Complete the TODO segments in each method. First, finish the constructor for Asteroid
according to the instructions. Then, finish the accessors for the x
, y
, and radius
members. Finally, complete the update()
method so that it updates the location of the asteroid. As with the Ball
class, update the x
and y
members based on their velocities multiplied by time.
Ship
Paste the code from Ship.java
into your Ship
class file.
Complete the TODO segments in each method. First, finish the constructor for Ship
according to the instructions. Then, finish the accessors for the alive
member. Most of the work in this class is in the collides()
method. In this method, you need to determine if any asteroid in the given array collides with the ship. Loop through all of the asteroids and compute the distance to the ship, using the standard Euclidean distance formula. If the distance from any asteroid to the ship is less than the radius of the ship plus the radius of the asteroid, return true
. If you get through the entire list of asteroids and none of them collide, then (and only then) return false
.
Simulator and StdDraw
For both the Simulator
and StdDraw
classes, simply paste in the code from Simulator.java
and StdDraw.java
.
Game Play
Once you have completed all the changes, you should be able to play the limited version of Asteroids. By simply moving your mouse, the ship will accelerate toward the mouse pointer. Try to stay alive as long as possible!
The instructor will give an demonstration of what the game should look like when the lab begins.
Turn In
Turn in your code by uploading Asteroid.java
and Ship.java
from the Lab12\src
folder wherever you created your project to Blackboard. Do not upload the entire project. The Simulator.java
and StdDraw.java
files are unnecessary. I only want the Asteroid.java
and Ship.java
files.
All work must be done individually. Never look at someone else's code. Please refer to the course policies if you have any questions about academic integrity. If you have trouble with the assignment, I am always available for assistance.