Lab 10: Sierpinksi Triangle
Due by the end of class
The Sierpinski Triangle is a fractal image made out of infinitely repeating triangles. Below is an animation going through the construction of a Sierpinski Triangle.
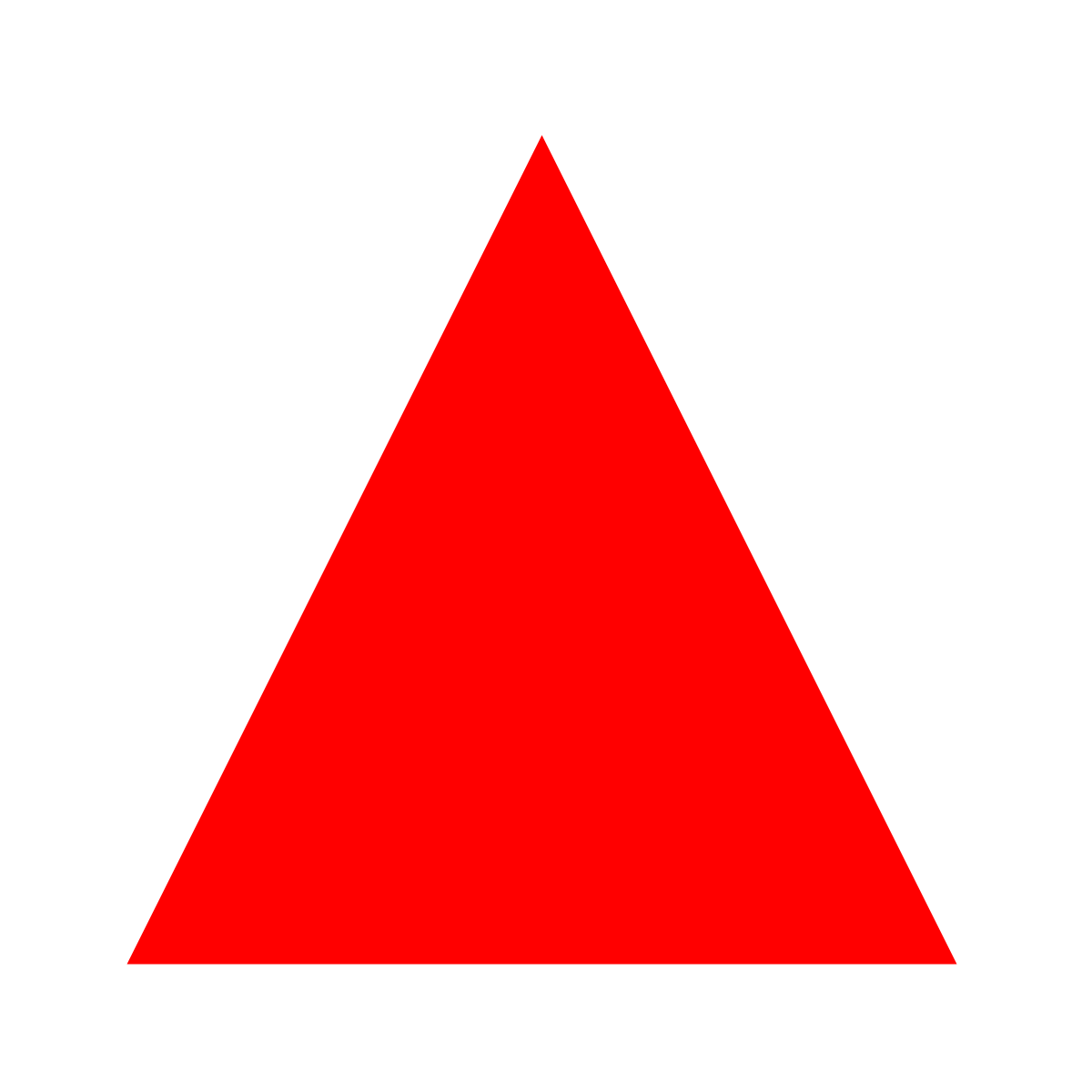
There are many ways to create a Sierpinski Triangle. One of the most unexpected is called the Chaos game. To play the Chaos game, you do the following:
- Pick 3 points in a plane to form a triangle.
- Randomly select any point inside the triangle.
- Move half the distance from that point to any of the 3 vertex points.
- Plot the current position.
- Repeat from step 3.
Amazingly, with only a few stray points, this process will draw a Sierpinski Triangle. We are going to automate this process using Java
Specification
Create a project called Lab10
. Add a class called Sierpinski
. Please try to spell "Sierpinski" correctly. Otherwise, automatic grading becomes much more difficult for me. Then, add another class called StdDraw
. Inside StdDraw
, delete all the code, and paste in the code from here.
First, prompt the user for the number of iterations he or she wants to run the Chaos Game for. Below is sample output for a user who has decided to run 1,000,000 iterations.
Please enter the number of iterations of the Chaos Game: 10000
You should allocate 3 pairs of x and y values, of type double
. Initialize the first pair to (0,0), the second to (1, 0), and the third to (.5, .5√3).
Next, you need to allocate variables for the current x and y location.
Now, you simply play the Chaos game described above. For simplicity, instead of picking randomly, pick (0, 0) as your starting point. Then, randomly pick one of the 3 corners of the triangle (either (0,0), (1,0), or (.5, .5√3). Use Math.random()
to get a value in the range [0,1). With a random number between 0 and 1, how can you make a uniformly distributed random decision about which corner of the triangle to move toward?
Once you have picked the corner, make the x value of your new point be the average of the old x value and the x value of the corner you have chosen. Make the y value of your new point be the average of the old y value and the y value of the corner you have chosen. Then, mark the point using the StdDraw.point()
method. Repeat this process as many times as the user specified.
Sample Output
Below is sample output from a program after it has run 10,000 iterations. Your output should match as closely as possible, though there will be slight variations due to the random nature of the Chaos Game. Note also that it will take a while for all iterations to complete, particularly if you run to a million or more.
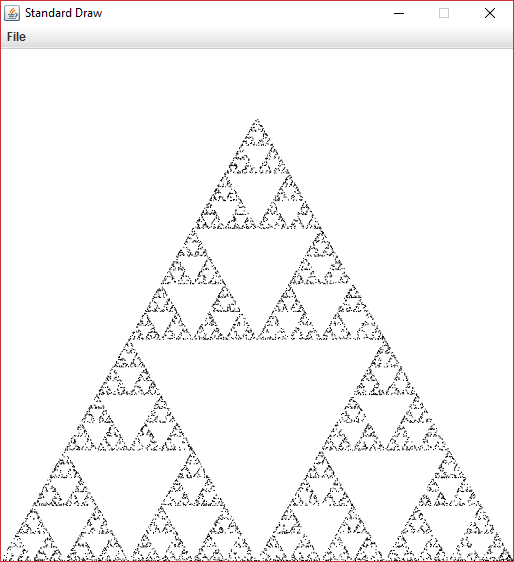
Turn In
Turn in your code by uploading Sierpinski.java
from the Lab10\src
folder wherever you created your project to Blackboard. Do not upload the entire project. I only want the Sierpinski.java
file.
All work must be done individually. Never look at someone else's code. Please refer to the course policies if you have any questions about academic integrity. If you have trouble with the assignment, I am always available for assistance.