Assignment 3: There's a box in the sky through which things can fly
Due by: Monday, October 16, 2023 at 11:59pm
Assignment 3 is a programming assignment intended to help you with some of the issues in Project 2 and also to prepare you better for Project 3.
Specification
The goal of this assignment is very simple: Create two textured cubes. Both cubes start centered at the origin (0,0,0). The first cube is small and should be textured with a photograph of your face on each of its six faces. The second cube is a skybox, very large with surfaces facing inward, rather than outward. You should also implement basic camera controls to move backwards, forwards, left, right and rotate the direction that is considered forward in both x and y. A screenshot from my implementation is below.
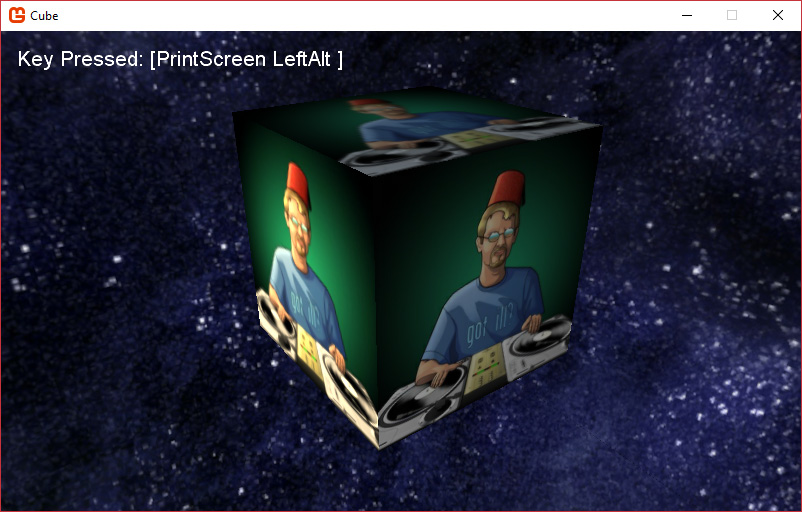
Photo Cube
I recommend that you base this assignment off of the simple polygon vertex buffer example we did in class. For the smaller cube, you will need to supply a photograph of yourself and little more, ideally in a power of 2 sized image. Feel free to use Pixlr to resize your images. I recommend that you make the cube have a "radius" of 5 units, stretching from (-5,-5,-5) to (5,5,5). You can think of this as 10 units on a side, but I think it's easier to think of it in terms of half-sides.
Skybox Cube
The skybox cube will be similar to the other cube, but each of its faces will have normals pointing the opposite direction. I recommend a "radius" of 100 for the skybox. Your program should work with culling turned on. The original cube uses a clockwise winding order. You can either create new set of vertices, set the culling to use a counter-clockwise winding order, or use a transform to flip the cube inside out. You can change culling modes by setting GraphicsDevice.RasterizerState
to RasterizerState.CullCounterClockwise
or RasterizerState.CullClockwise
.
You may create your own skybox texture or use the one I provide here. I apologize for the size of this texture, but it's hard to find something that looks good at a smaller size. Most of the difficulty of this assignment is matching up the correct face of the cube with the right part of the texture, oriented the right way. Even if you copy the vertices from the original cube, you will have to set new texture coordinates for them. I don't care which part of the texture is the front, back, left, right, top, and bottom, but the skybox should be seamless.
Lighting
A standard directional light is fine for the cube. Try diffuse and specular colors of (.5, .5, .5) and a direction that hits at least two faces like (√2/2), 0, -√2/2). Feel free to experiment with other directions.
The skybox should be lit differently, since it represents the sky. Its lighting should be completely even and unchanging. The best way to manage this is to turn lighting off before rendering the skybox.
Camera
Start your camera at (0,0,50), looking at the origin.
You must support basic camera motion:
- W - Moves the camera forward
- A - Moves the camera left
- S - Moves the camera backward
- D - Moves the camera right
- Left Arrow - Rotates the forward direction counterclockwise around the y-axis
- Right Arrow - Rotates the camera clockwise around the y-axis
- Up Arrow - Rotates the camera clockwise around the x-axis
- Down Arrow - Rotates the camera counterclockwise around the x-axis
The trick to making this work is defining forward as (0, 0, -1), right as (1, 0, 0), and up as (0, 1, 0). Keep track of the translation of the camera and the rotation of the camera. When you rotate with the arrow keys, use an Euler transform (using the Matrix.CreateFromYawPitchRoll()
method) to rotate forward, right, and up directions based on the rotation of the camera. Once you have transformed forward and right, you can add (scaled) versions of those to the translation of the camera corresponding to W, A, S, D key presses.
Once you have the translation updated for the camera, you still have to use the Matrix.CreateLookAt()
method to set where it looks. The position is taken care of. The look at location can be found by adding the forward vector to the position. Finally, the rotated up vector (which you haven't used yet) is the new up vector for the camera.
Note that the skybox should not appear to move in order to appear to be at infinite distance. Thus, its world translation must change to keep up with the changing camera translation.
The projection transform is not greatly different from other projects and assignments. The only thing you need to be careful of is keeping the skybox within viewing range. A near plane at 1 and a far plane at 1000 should work fine.
Sample Implementation
You can download a sample implementation here to get a feel for how my code works. You don't need to match it exactly.
Turn In
Zip up your entire project and solution. Upload that zip file to Blackboard. All work must be submitted by Monday, October 16, 2023 at 11:59pm. Grace days are not available for assignments.
All work must be done individually. You may discuss general concepts with your classmates, but it is never acceptable for you to look at someone else's code. Please refer to the course policies if you have any questions about academic integrity. If you have trouble with the assignment, I am always available for assistance.
Grading
Your grade will be determined by the following categories.
Category | Weight |
---|---|
Photo Cube | 25% |
Skybox | 30% |
Lighting | 15% |
Camera Movement | 30% |