Assignment 2: Turtle Power
Due by: Friday, September 8, 2023 at 11:59 p.m.
In this assignment, you'll use the turtle module to practice your skills at writing functions and using for loops. Make sure you've read chapter 1 carefully, especially the introduction to the turtle module in section 1.5.
drawLine() function
The first step is to implement a drawLine() function. It should move the turtle forward but then also return the turtle to its starting position. The first argument is the turtle object, and the second is the length of the line.
The function should have the following header:
def drawLine(myTurtle, distance):
drawRays() function
Next, implement a drawRays() function. It should draw a starburst pattern of rays emanating from a single point. The rays are evenly distributed around the central starting point. The first argument is the turtle object, the second is the number of rays, and the third is the length of the rays. For example a new turtle will produce the following in response to drawRays(myTurtle, 10, 100):
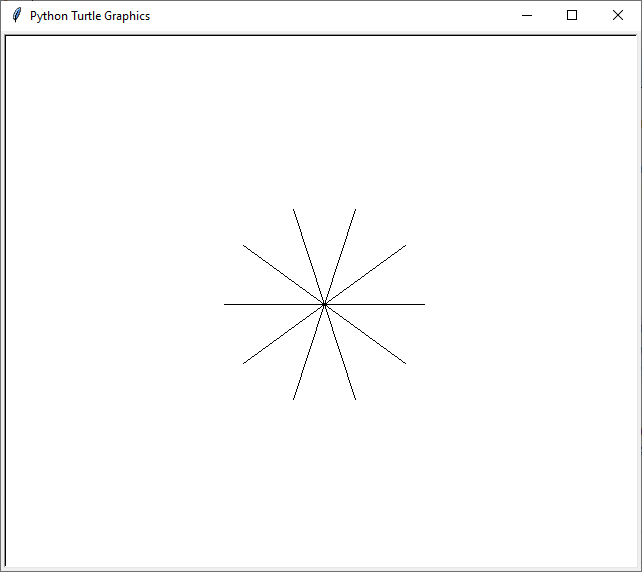
The function should have the following header:
def drawRays(myTurtle, lines, distance):
Hint: You will need a for loop for this function, and you will also use the drawLine() function you created before.
Drawing many stars
Implement a new function that makes multiple calls to drawRays(), producing output similar to this (duplicate it as closely as possible):
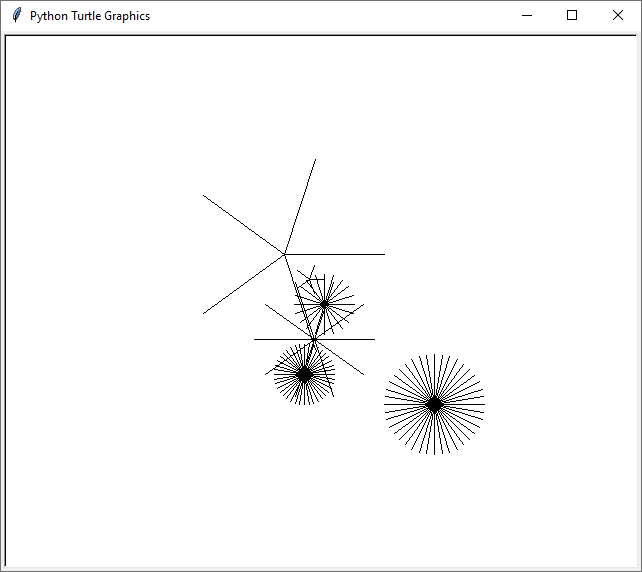
Save your final code that draws this cluster of starts in a file called assignment2.py. This code should run without error and draw the desired stars.
Turn In
Upload assignment2.py to Blackboard.
All work must be submitted before Friday, September 8, 2023 at 11:59 p.m. unless you are going to use a grace day.
All work must be done individually. You may discuss general concepts with your classmates, but it is never acceptable for you to look at someone else's code. Please refer to the course policies if you have any questions about academic integrity. If you have trouble with the assignment, I am always available for assistance.
Grading
Your grade will be determined by the following categories:
Category | Weight |
---|---|
drawLine() function | 20% |
drawRays() function | 30% |
Code to draw all stars | 50% |
Under no circumstances should any student look at the code written by another student. Tools will be used to detect code similarity automatically.