COMP 325 Lecture 11: Object Oriented Analysis: Objects
and Associations
major resources: Introduction to Object Oriented Analysis 2002,
Object-Oriented and Classical Software Engineering 6ed,
Schach 2005, Object-Oriented Software Engineering, Schach 2008.
[ previous
| schedule
| next
]
Object Oriented Analysis (OOA)
Major advantage of OOA: ability to create in the computer world things that accurately reflect the things your users work with in their real world. (Brown, p 100)
- Object in real world: a thing, capable of being seen, touched, or otherwise sensed.
There are many such things, we need to abstract away the ones not relevant to the task or project that we are analyzing.
- Object in the data world: representation of a real-world object.
An abstraction of its description and behaviors.
- Some additional objects with no real-world analogue may be needed; we'll come back to this shortly.
- Ideally, the representation developed in analysis can be refined and used throughout development (design, implementation, testing, maintenance).
- This results in seamless transitions among phases and enhanced traceability, important for validation and maintenance.
Classification of objects
Ivar Jacobson, one of "The Three Amigos", classifies objects into 3 types which were adopted into UML:
- Entity objects
- named after the Entity in ER diagrams
- represent real world objects
- have properties and behaviors of interest to clients
- not all take physical form; some are more conceptual
- Boundary objects (originally called Interface objects)
- Objects that communicate between system objects and external entities.
- The external entities can be human or electronic
- The desire is to localize the effects of changes in external environment.
- GUI widgets are an example, APIs are an example, network communication is an example
- Control objects
- Objects that carry out behaviors involving data from several different classes of objects.
- these are usually complex algorithms
- Such behaviors do not fit neatly into entity or interface objects.
Consider advantages of organizing objects into these categories
- Improves system stability because important core objects are not affected by changes in entities external to the system
- Facilitates maintenance because changes are more localized.
- Makes system more flexible because it can be adapted more easily to new external environment
These relate to Model-View-Controller (MVC) pattern
- Originally developed for Smalltalk-80
- What do the model, view, and controller do?
- Model: models state and behavior of system entity
- View: determines how model will be displayed
- Controller: handle user input
- One way for them to interact follows the Observer Pattern
- View registers as observer of model
- Controller registers as listener of input object
- When controller is notified of input event, it changes the model
- When model changes, it notifies the view(s)
- When view is notified of model change, it changes its display
- To do the preceding in Java,
- the model itself is of a class that extends the Observable class,
- view is of a class that implements the Observer interface, and
- controller is of a class that implements the ActionListener interface
- controller must be registered as an action listener of GUI input component
- a more limited variation does not use observers
- Controller registers as listener of input object
- When controller is notified of input event, it changes the model then the view
- The observer pattern is more powerful than the simpler variation:
- view will change regardless of
what triggered the model change. It could have been triggered by another method in the
model. The variation can change the view only as direct consequence of user input event.
- additional views can register without affecting model or controller code. The variation
requires additional code in the controller to handle the additional view.
Schach OOA Process
The goal is to find and record classes, their attributes, behaviors and relationships.
Schach promotes an iterative and incremental process for extracting entity classes
- Describe scenarios, or instances of use cases. This is called functional modeling
- From scenarios, determine entity classes, attributes, interrelationships and express results in
class diagram. This is called entity class modeling.
- For each class, determine its operations or behaviors and express results in statechart.
This is called dynamic modeling.
Boundary and control classes are then ("easily") found; boundary classes correspond to input and output screens
and reports, control classes correspond to "non trivial computations".
Use cases are refined, then "realized" through collaboration and/or sequence diagrams to model system dynamics.
Brown OOA Process
The goal is to find and record classes, their attributes, behaviors and relationships.
Brown promotes the 7-step KRB (Kapur-Ravindra-Brown) process
- discover candidate classes
- define classes based on candidate lists
- establish associations between classes
- expand M-M associations into 1-M
- find attributes
- normalize
- find behaviors
These are outlined in detail, below the section on Responsibility-Driven Design.
Artifacts of the OO Analysis Workflow
Artifacts produced by the OO Analysis workflow include:
- refined use case diagrams
- refined use case descriptions
- use case scenarios (if distinguished from descriptions)
- class diagrams
- statechart diagrams
- collaboration and/or sequence diagrams
- UI prototypes possibly
These artifacts and the processes that produce them are described over the next two lectures.
Responsibility-Driven Design and CRC cards in OOA
- RDD developed in late 1980s, principally by Rebecca Wirfs-Brock, see http://www.wirfs-brock.com/
- can be used as alternative to or in conjunction with, use cases and statecharts
- is not part of UML
- Goal is to build collaborative model of application by defining a "community
of objects" and assigning specific responsibilities to each (paraphrased
from Wirfs-Brock Assoc homepage)
- can be applied in either a primary or secondary role in analysis or design workflow
RDD Concepts
- objects have responsibilities: the services it provides
- objects enter into contractual client-server relationships
- an object may collaborate
with (get assistance from) a different object to complete the service and fulfill its responsibilities to its client
CRC Cards
- Class-Responsibility-Collaboration (CRC) cards can be used to identify a
class's responsibilities and collaborations
- CRC cards developed by Ward Cunningham and Kent Beck, pioneers of the agile development movement, in late 1980s
- these are literally index cards, with 4" x 6" most favored (if filled, class is too large)
- in essence:
- each card represents a class
- a list of responsibilities is compiled interactively while walking through
use cases
- each responsibility can have a list of classes with which the object
needs to collaborate to complete the responsibility
- some collaborations can be identified and listed during the walk-through
- at the end of the walk-throughs, each class (card) will have a
list of responsibilities and possibly collaborations.
- responsibilities include the things it knows (data)
as well as the things it does (behaviors)
Walk-through of use cases
It is useful to conduct walk-through of use cases to identify and record responsibilities and collaborations
- one person plays the user, the rest play classes
- the persons representing classes hold CRC cards for their class(es)
- the person representing the user reads (aloud) steps from use case
- for each step:
- user asks appropriate class if it can provide the needed service/data
- if not, then class should add responsibility to its list and check for
collaborators (other classes)
- if collaborator(s) found that can help out, collaboration class is listed
next to responsibility
- new classes may also arise from this exercise
- repeat until all use cases covered
Details of KRB method of OOA
Step 1: discover candidate classes (entity, boundary, and control)
- Discovering Entity classes - client can relate most directly to these
- Use one or combination of the following techniques
- interviews with clients
- get from Requirements or other docs, particularly nouns in use case descriptions
- interactive brainstorming session - generate ideas, then evaluate them in Step 2.
- "Delphi" method: get lists of candidate classes from individuals, then
combine and distribute for comment
- Discovering Boundary classes
- for user interface, use GUI class library
- for communications interface, use established protocols
- for interface with external system, use documentation from that system
- Discovering Control classes
- recall this is class created to handle operations required many other
classes
- more critical in design phase, since control classes are usually not
tangible in user's world
Step 2 : define classes based on candidate lists
- Purpose is to narrow list of candidate classes to only those of interest
- Method is to subject each candidate class to 3 checks:
- real-world identifier
- can you establish a distinct identifier for each object of this class?
(i.e. can you tell them apart?)
- if, so it may be significant class
- record your findings
- definition
- can you define what an object of this class is? (i.e. can you answer
the question "What is a <insert candidate classname here>?")
- if competing definitions arise, may require two or more unrelated classes
- if struggling to come up with definition, class may not be significant
- may give clues to superclasses (e.g. describing a bus as a kind of vehicle
for ...)
- The terms and definitions need to be recorded to build project dictionary
- sample attributes and behaviors
- can you describe attributes for this class that are relevant to the
system? ("What do I need to know about a <class>?")
- can you describe behaviors for this class that are relevant to the system?
("What relevant things can a <class> do?" or
"What can I do with an object of <class>?")
- if you can describe either, then it is probably significant
Step 3 : establish associations between classes
- association: "relationship expressing interaction between instances
of two classes"
- Discover verbs
- see if you can find a verb that describes what one object can do to
another
- or see if you can find a noun that describes the role
that one plays in the life of the other
- make sure that the association makes sense in the user's world and vocabulary
- matrix method for methodically discovering and recording associations among N classes
- draw an N x N matrix, with classes listed both as rows and columns,
- check each cell for association
- annotate cell with discoveries or at least check it off to indicate
you've explored it
- establish cardinality
- once association established, determine how many on each end.
- use class names and association name to ask How many <receiver class>
does a <sender class> <association>?
- e.g. if the classes are vender and product and the association is sell,
the question is "How many products does a vender sell?"
- Sometimes this discussion leads you back to Step 2 definitions, and
possibly to clarification or modification of definitions.
- It is sometimes difficult to determine if an association should be 1:M
(one-to-many) or M:M (many-to-many).
- Example: A vendor sells many different products, but is a product
sold by one vendor or is it sold by many?
- If the former, the association is 1:M; if the latter, M:M.
- Rule of thumb: Is each product object
uniquely identified (e.g. by a serial number)? If so, the association
is 1:M; if not, M:M.
- UPC codes don't count as unique identifiers because they identify
product types, not individual product objects. Some products
such as cars definitely have unique identifiers and are thus sold
by one vendor in a transaction.
Step 4 : expand the M-M associations into 1-M
- M-M means many-to-many association, 1-M means one-to-many association
- 1-M are much easier to implement in a database than M-M.
- 1-M modeled using foreign key (relational DB term:
a field whose value is a key in different DB table. NOTE: OODB uses pointer instead)
- example of 1-M is library patron and books checked out, assuming each book has unique ID
- a patron can check out many books and a book can be checked out by only
one patron
- a database table BooksCheckedOut can represent the class of those
objects (books checked out)
- a database table Patrons can represent the class of those objects
- each entry in the Patrons table has a unique key field PatronNumber
- each entry in the BooksCheckedOut table has a PatronNumber
field to store the ID of the patron who has checked out this book - the foreign key
- For any record in the BooksCheckedOut table, we can find information
about the corresponding patron
- For every record in the Patrons table, we can find information
about any books this patron has checked out.
- M-M is more difficult
- Example of M-M is students and sections.
A student can register for many sections,
and a section can have many students registered
for it.
- Student database one record per student, section database one record
per section
- one solution strategy, which we reject, is for either student record
to have list of section keys or section record to have list of student
keys, or both.
- This is comparable to the foreign key solution above.
- Reject because it violates First Normal Form (see Step
6 below) - field should contain only one value
- Better solution is to:
- define intermediary, intersection class called registration.
Represents event of student registering for a section.
- establish 1-M relation between both of the existing classes with
the new intersection class.
- Each section has many registrations,
each registration has only one section.
- Each student has many registrations,
each registration has only one student.
- Each registration record (object) has two foreign keys: one for student,
one for section.
- The general resolution to M-M problem:
- define third, intersection class
- define 1-M association between the original two classes and the new
one
- for both 1-M associations, the M end is at the intersection class
Step 5 : look for attributes
- For each box (class), brainstorm on attributes
- list attributes in or next to box, if room
- as each attribute is listed try to define it
- key attributes, those whose values uniquely identify the object, should be marked with asterisk (*)
- key may involve more than one attribute
Step 6 : normalization (adapted from DB theory)
- Brown gives simple intuitive definition: normalization is ensuring that
every attribute is attached to the class it truly describes
- also reduces redundancy
- reminds me of single point control principle ("define once,
use many times")
- our examples have relational database flavor
- Think of each table (grid) as representing a class
- think of each record (row) as representing an object
- think of each field (column)as representing an attribute
- translated to relational database terms, normalization is ensuring that
every column is in the table that it really pertains to
- normalization has several levels, each of which includes the previous. We'll look at first three:
- First Normal Form 1NF
- Second Normal Form 2NF
- Third Normal Form 3NF
- If your system is in 3NF, it will be quite flexible and resilient to change.
- The tradeoff is possibly reduced performance.
- First Normal Form (1NF)
- no table has repeating fields in a record
- e.g., no list of books checked out by library patron
- using fields for lists fixes the maximum list length, which is not flexible/resilient.
- OO dilemma of multi-valued (non-primitive) attributes
- Step 4 put the classes into 1NF by resolving M:M to 1:M
- Second Normal Form (2NF)
- all non-key fields require the whole key (they are fully
functionally dependent on the whole key)
- put another way, if there are fields that only require partial key,
it is not in 2NF.
- back to example of students and sections: The intersection
table, registration, has a 2-part key, student ID plus section
ID. Suppose it also had a non-key field called "room" for
the room number of the class. The "room" value can be determined
from a partial key (section ID only) and it would not be in 2NF.
Resolve this by moving "room" into the table that it depends
on, section.
- Third Normal Form (3NF)
- "everything depends on the key, the whole key, and nothing
but the key" (Brown, p. 326)
- i.e. there are no non-key fields that can be determined by other non-key
fields
- this is called transitive functional dependency
- This addresses the phrase "nothing but the key" (2NF
addresses "the whole key").
- back to the example of students and sections. Suppose the
section table had fields for building number and building name.
The building name can be determined given the building number and vice
versa, it is not in 3NF (non-key field determined by another non-key field).
The solution is to define a building table containing both number and
name, then remove the building-name field from the section table. This
creates a new class for buildings (remember that table == class)
- summary of putting into 3NF:
- find non-key field that can be determined by another non-key field
- create new table (class) with those two fields and eliminate dependent
one from original table
- repeat until no more such fields exist
Step 7 : find behaviors
This involves discovering and specifying system dynamics. These topics are explored
and described in the next lecture.
Class Diagrams
Class with attributes and behaviors annotated with visibility |
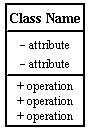 |
Entity class (stereotype - not standard UML) |
 |
Boundary class (stereotype - not standard UML) |
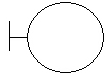 |
Control class (stereotype - not standard UML) |
 |
Generalization association |
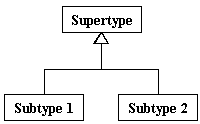 |
Composition and Aggregation associations
(composition is like engine to car, dependent)
(aggregation is like book to shelf, independent) |
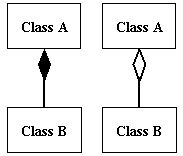 |
general associations |
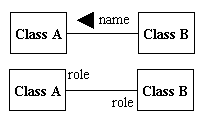 |
cardinality (multiplicity) |
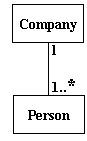 |
[ COMP 325
| Peter Sanderson
| Math Sciences server
| Math Sciences home page
| Otterbein
]
Last updated:
Peter Sanderson (PSanderson@otterbein.edu)