COMP 2100 Project 1: Implementing a statistic class
(20 points)
Due: in lab, Thursday September 3, 2015
You are to implement a Statistic class based on its published
specification. You can think of a Statistic object as "an observable random variable" (Wikipedia).A client should be able to use this class to do a number
of things: create a named Statistic object, collect a numeric value,
retrieve the smallest or largest value collected so far, retrieve the sum, mean
(average) or standard deviation of the values collected so far, retrieve a count
of the number of values collected, and retrieve its assigned name.
Here is an example of client code that use this class:
public static void main(String[] args) {
Statistic scores = new Statistic("quiz scores");
scores.collect(5);
scores.collect(7);
scores.collect(2);
scores.collect(0);
scores.collect(1);
System.out.println( "Number of scores: " + scores.count() );
System.out.println( "Highest score: " + scores.highest() );
System.out.println( "Lowest score: " + scores.lowest() );
System.out.println( "Average score: " + scores.mean() );
}
This program will produce the output:
Number of scores: 5
Highest score: 7.0
Lowest score: 0.0
Average score: 3.0
Details
- Download the project zip file and unzip (right-click, Extract All).
It will create a pr1 folder containing
- StatisticDriver.java, a client class that will thoroughly test your solution.
- Statistic.java, where you will define your solution. It is an empty class.
- pr1.gpj, a jGRASP project file.
- Launch jGRASP and from the Project menu, select Open. A dialog will appear. Browse to your pr1
folder and select pr1.gpj.
- Notice that an Open Projects section is displayed in the Browse tab (left center of the screen).
I have already created a Project file and added both StatisticDriver.java and Statistic.java to the project.
They are listed here and you can open them from here.
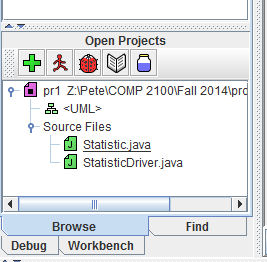
- Open Statistic.java and implement the methods as specified. I recommend
that you start by writing the methods as stubs. This means they will
have their correct return type and parameters, they will compile, but they
will not do anything. For void methods (commands), the method body
will be empty - nothing between the curly brackets. For methods that return
a value (questions), the method body will have to contain a return
statement that returns a dummy value of the expected type - e.g. return
0.0; if the return type is double.
- You need to decide what instance variables will be needed to implement these
methods, and add them to the class definition. They should of course be private.
- Do NOT store every collected value, such as in an array. You don't know how many there will be. All methods,
including standard deviation, can be implemented without storing individual
collected values. Explanations follow.
- Given a collection X of values x1, x2, ..., xn,
the mean is defined as (Σ xi)/n. Think about how you
can calculate this without having to keep all the xi.
- The standard deviation of a population of values is defined as the square
root of the variance of that collection. Variance is defined as the average
squared deviation from the mean. The formula to calculate variance
can be given as: (Σ xi2)/n
- ((Σ xi)/n)2, where xi are individual
values collected and n is the number of values collected. For example, if
you collected the values 1, 2, 3, 4, 5, then (Σ
xi2)/n =
(1+4+9+16+25)/5 = 55/5 = 11, and ((Σ xi)/n)2 =
((1+2+3+4+5)/5)2 = (15/5) 2 = 32 = 9, so the variance is 11-9 = 2. The standard
deviation is the square root of this, which for this example is 1.414.
- Due to inaccuracies in computation with floating point numbers, operations
on the Java double data type
do not throw exceptions (for such conditions as zero divide); they instead
produce a special constant result called Double.NaN.
In the situation where no values have been collected yet, count()
should return 0 and sum() should
return 0.0, but the others (highest, lowest, current, mean, standardDeviation)
should return Double.NaN.
- Best Solution Approach: Do all calculations and update instance variables in
the collect method, since this is where a new value comes in. By
doing so, the other methods (highest, lowest, etc) can just return the updated
value of the appropriate instance variable.
- In addition to all this, document your code using Javadoc-style comments.
Such comments should go at the top of the class and
before each method. Your methods should use the @param
and @return tags as appropriate.
- With the Project open (see items 2 and 3 above), you can select the Documentation
icon (the open book) -- or Generate Documentation from the Project menu -- to generate web pages from the Javadoc. A dialog will appear.
Uncheck the "Include private items" box, and click Generate. jGRASP will create a folder called pr1_doc
in with your other files, and generate all the web pages into that folder. A browser window should pop up with
the index.html page displayed. Every class in the project is included, so to see the Statistic web page
click its name in the upper left.
- If the previous step fails, and it probably will, refer to the document
Generating an API webpage from a Java source file with Javadoc-style comments for
instructions on generating the API using a Windows command prompt.
Scoring
Points | Item | Description |
3 |
documentation |
Use of comments including identifying (include your name!!) and Javadoc-style comments, use
of self-documenting identifier names, and judicious use of white space such
as indentation to enhance readability. |
17 |
results |
The constructor, name, and current are worth 1 point each. All other
methods are worth 2 points each. Even though the calculations are done in collect(), the points will
accrue to the methods that return the result of those calculations. |
To Turn In
Drop Statistic.java into your COMP 2100 DropBox folder on the I drive.
[ COMP
2100 | Peter Sanderson
| Math Sciences home page
| Otterbein ]
Last updated:
Peter Sanderson (PSanderson@otterbein.edu)